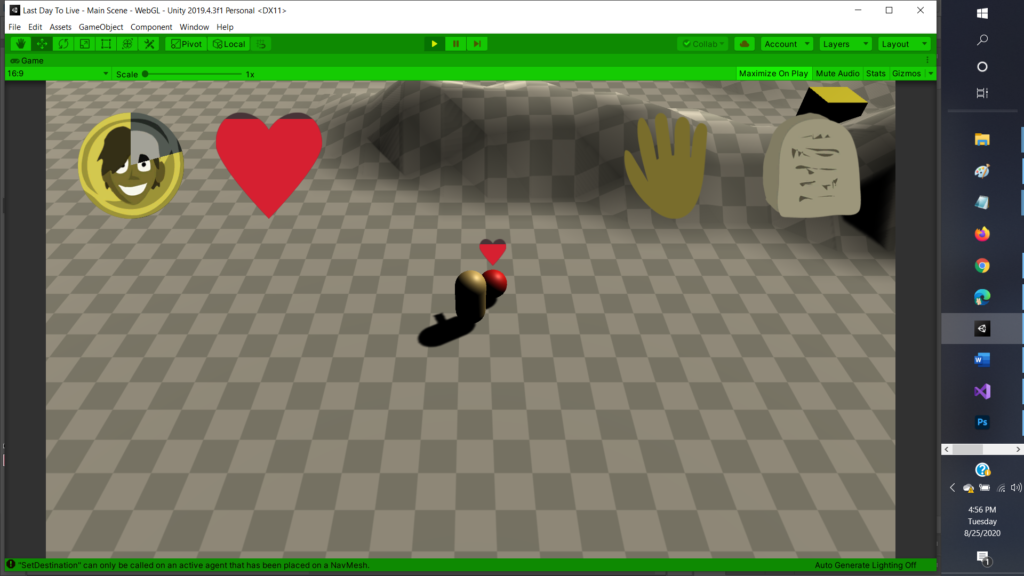
So, I’ve made some progress on Last Day To Live since my last post. I got through the Unity Learn Beginner Programming course, and used what I’d learned there to redo the player control code using a state-based machine, which made the code a lot cleaner… and made it much easier than it would have been to add the ability for the player to attack enemies.
I have once again put the latest build online; you can play it, if you’re so inclined, here.
By the way, I had some trouble building the game; I kept getting the error “cannot build player while editor is importing assets or compiling scripts”. A web search showed that this error came about if any of the scripts had a “using UnityEditor” directive. Well, I certainly hadn’t intentionally included such a directive, but I had experienced before that sometimes Visual Studio’s autocomplete would put in something from a namespace you hadn’t been using yet, and would add the appropriate using directive itself, and I figured that was what had probably happened. Sure enough, it turned out that for some reason the PlayerJumpState script had the line “using UnityEditorInternal”—not quite the same as “using UnityEditor”, but apparently it caused the same issue, because when I removed it the build proceeded just fine. Now, this feature of Visual Studio’s autocomplete had annoyed me before, and I figured it was about time to finally find a way to disable it—sure, it could sometimes be helpful, if it added a using directive you did want but had forgotten, but it added unwanted using directives and incorrect autocompletes often enough to make its vexatiousness overshadow its usefulness. Turns out this feature could be easily turned off in the Visual Studio options (accessible through the Tools menu), and for anyone else who might be as annoyed by this feature as I am, here’s how to disable it:
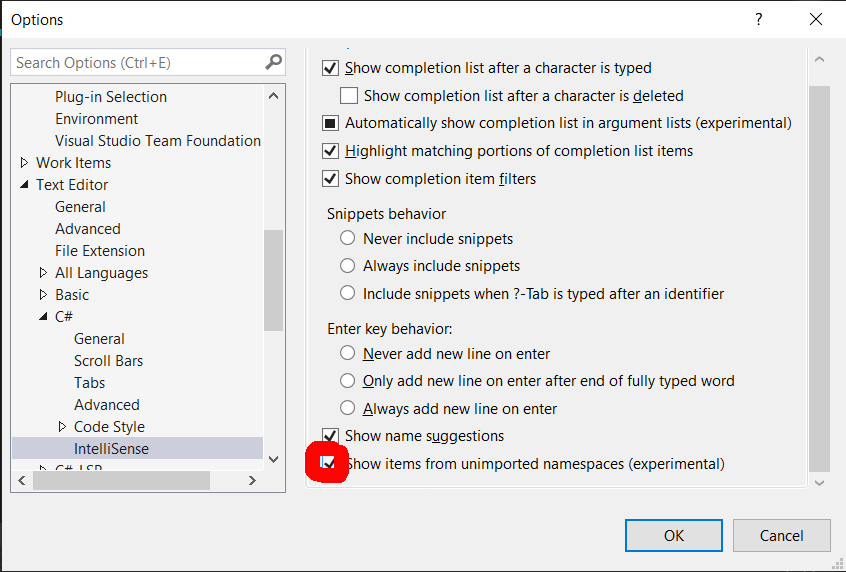
Mind you, there are still a few bugs to work out with the combat functionality in Last Day to Live, and the fact they only happen occasionally and aren’t easily reproducible makes them all the harder to track down. Sometimes the player will run not just toward but past an enemy and then keep backing away from the enemy as he fights—and clicking on the enemy again will make the player fall through the ground! Sometimes the player will run up to an enemy and seem to be fighting, but not actually inflict any damage. I also just realized there’s an issue with the regular movement code, in that if the player clicks on the ground while in the middle of a jump then the navigation won’t kick in like it should “because it is not close enough to the NavMesh”, as the error message in the console puts it—I think I know how to fix that, but I haven’t gotten to it yet. Anyway, I’m definitely going to try to get rid of these bugs, but I didn’t want to wait until that was done before making this post because, well, this post is a few days later than I’d planned as it is. It’s been a busy week—work is starting to pick up again, which is good from a financial perspective, but maybe not so good from a free time perspective. (Still, ultimately not being able to pay bills would end up cutting into free time, since it would be hard to get anything done without food or a place to live, so overall this is a good thing.)
As you can see from the screenshot above, I’ve also started to implement the UI. The two icons on the right are for inventory and the main menu, but they don’t do anything yet. The two on the left, however, are fully functional; the leftmost icon shows the time until the player is killed by the curse, and the one on the right shows the player’s current health. These icons are definitely not final versions; I made some quick placeholder UI graphics for now, but I intend to replace them with more polished graphics later—though very possibly not before the Graduation Play-a-thon.
The time icon took a bit of trickery to get it to work the way I wanted, with a healthy face doing a sort of a “clock wipe” into a skull. There may have been an easier way to implement it, but the way I ended up doing it involves four different images stacked on top of each other—two of the face and two of the skull—, with rotating masks on the top three images. I hadn’t used UI masks before, so that’s one new thing I learned about while doing this (after doing quite a bit of websearching and looking through the documentation to figure out how to do what I wanted).
When it came time to fully implement the combat system and allow the player and the enemies to actually damage each other, it occurred to me that I’d never actually decided how I wanted the damage to be shown. Did I want numerical damage values to appear above the enemies? Did I want the enemies to have health bars like in the Create With Code Prototype 2 Expert bonus challenge? Ultimately I decided to give the enemies hearts to indicate their health like smaller versions of the heart indicating the player’s health in the UI. I also added a few more classes to generalize the necessary behavior between the enemies and the player and make it easier to create more objects later that shared aspects of that behavior—the player and enemies now both derive from a class called Combatant, which in return derives from a class called Damageable (which I’ll be able to use later if I want to implement things that can be damaged but can’t fight back, like smashable barrels). There are also eight scripts for the player’s behavior: the main PlayerController script, an abstract PlayerBaseState class for the classes for each of the player’s states to derive from, and then classes for each of the player’s six current possible states: Idle, Move, Nav, Attack, Jump, and Dead. (The Move state is for when the player moves directly toward the cursor when the mouse button is held down; the Nav state is for when the player uses the NavMesh to navigate toward a point.) There’ll ultimately be more states I’ll add later: a Talk state for when the player is talking to an NPC, an Interact state for when the player is taking or interacting with an item—but the state-based structure of the code is going to make those relatively easy to implement. Together with the GameManager, the EnemyScript, and various scripts to manage the UI, the project now contains a total of seventeen scripts, and that number will of course increase as I add more features, but of course that’s not a bad thing; a lot of small, specialized scripts are easier to maintain and debug than a few gigantic scripts that do everything.
So, anyway, I guess at this point I’ve more or less got a (Very) Minimal Viable Product? I mean, the really core functionality is there. The player can fight enemies, can be damaged, and can die and be brought back to life (though not yet with any fanfare or explanation of why he’s brought back to life; he just reappears back at start with full health), and the game ends when the curse timer runs out. (The curse timer, by the way, runs out much faster now than it will in the final game—I put in a relatively short timer to make sure it was working, but in the finished game the timer will go a lot slower. I did say that the one day the player has left to live in-game will be shorter than a real-time day, but not that much shorter.) There’s no actual win condition yet, of course, but that’s… honestly way on down the line.
Unfortunately, I’m still a week behind where I ought to be by now according to my Project Design Document; by the schedule there I ought to have implemented interactable NPCs, and a scripted opening. So I guess that’s next on my list… sort of. The scripted opening won’t be what I’d originally had in mind; I’d thought of having some sort of cut scene where the player is killed by the main villain, and then is resurrected by a priestess character who explains what’s going on. But at this point I, well, haven’t really even decided who the main villain is, or why the villain curses the player in the first place, and anyway even if I do want to have such an opening cut scene in the final game (and I’m not 100% sure at this point that I do), it’s not something that needs to be or should be implemented this early on. I do still want to make a scripted opening of sorts, but just starting with the player being resurrected and the priestess explaining what’s going on—and putting the player through an optional tutorial to teach the control scheme.
I also realize that at no point in the timeline in my Project Design Document have I included implementation of items and inventory. Hm. I guess I should try to work that in, too, but maybe after the NPCs. According to my Project Design Document timeline, by next week I’m also supposed to have implemented more enemies, and “at least one simple environmental puzzle”. Well, the “more enemies” will be easy; now that I have the basic enemy code done, implementing variant enemies with different speeds, hit points, damage, and so on will be simple. (Eventually, of course, I’m probably going to want to give some enemies special abilities to make things more interesting—but that’s another thing that’s going to be further on down the line.) The environmental puzzle… maybe less so. We’ll see how it goes.
As for my learning Unity, like I said, I got through the Beginner Programming course, and the section on Finite State Machines has already proved useful for Last Day To Live—as has the section on the Character Controller and AI for implementing the enemy behavior (I think most of what was in that part had already been covered in other courses I’d taken, but it was still good to have the refresher), and I guess I put into practice a bit of what I took away from the section on the Observer Pattern for the interaction between the player and the UI. I don’t necessarily see any immediate application in my game for the Scriptable Objects described in the final section in the course, but it’s certainly possible I may find a use for them.
In the meantime, having finished that course, I’ve started another course on Unity Learn: Beginner Design. The first section of that course, Game Design Fundamentals, includes a template for a game design document far more in-depth than the one provided for the Create With Code course, though of course that makes sense; the personal project for the Create With Code course is intended to just be a minimal viable product for which such extensive design notes aren’t necessary. Still, if I intend to keep working on Last Day To Live after the course is over and to expand it into a complete game—which I do—then at some point I do need to plan it out in more depth, work out the details of the story, and so on. So I do plan to fill out the detailed design document, but not till after the end of the Create With Code course. The second section of the Beginner Design course, and the one I’m currently taking, is about level design, which I think may be my favorite aspect of game creation—I like all aspects of making games, and have lots of ideas for games I want to create more or less on my own, but if I had to pick one specific job in the game industry, I’d want to be a level designer. Now, Last Day To Live, as I conceive it, wouldn’t have discrete levels, per se; it would be more of an open-world game with a large area to explore. (Which means at some point I’m going to have to figure out just how best to implement such an open world, since I’m sure it’s impractical to have the whole world in memory at once and I don’t know the best techniques for dynamically loading the necessary parts of the world—I’ve found some tutorials about how to do this, but I haven’t really gotten into them yet; this is another thing that’ll wait till after the Create With Code class is over.) Still, many of the principles of level design apply to open-world games as well, besides which I have ideas for other games that will have discrete levels. Anyway, it’s interesting to learn more about the nitty-gritty of level design considerations and the relevant industry jargon.
Now, of course, there’s one big thing missing in Last Day to Live as it currently stands: graphics. Or, more specifically, models and textures. Everything is still done with flat-colored primitives. Whether or not I get this done by next week remains to be seen, but at some point before the Graduation Play-a-thon I do hope to replace these primitives with better models—not necessarily the final models I’ll ultimately use in the finished version of the game, but at least passable placeholders. I’ve been looking through the Unity Assets Store, and I’ve found free models I like for the enemies and the environment. The one thing I haven’t found models I like for, though, is the player—well, and human NPCs I’ll eventually add. I have a pretty good idea in my head for how I want the player to look, and I haven’t found any models in the Asset Store that remotely match it.
Well… I haven’t found any free models that remotely match it. Or models for less than $30, for that matter; I got desperate enough I figured that despite my current financial straits (thanks to the aforementioned slow work because of the COVID-19 situation), I could maybe afford to splurge a little. I did find one customizable character asset that looked like it should be able to make something close enough to what I wanted, a “Chibi – Male Pack 01” by Meshtint Studio, but it was priced at $49.90, which is a bit more than I feel I can comfortably afford at the moment—especially if I also wanted the matching “Chibi – Female Pack 01“, which I would, for female NPCs. That costs $39.90, so I’d be looking at a total of almost $90.00. (Why is the Female Pack ten dollars cheaper than the Male Pack? I have no idea. Possibly it has fewer models? The description of both packs just says they have “[m]ore than 300 game models”, and I don’t feel like counting the models in the listed package content to see. Or maybe the Female Pack wasn’t selling as well, so its creator dropped its price?) Now, as it happens, there was a “Discounts & Dragons” sale on fantasy assets in the Asset Store that just ended at midnight on Sunday, and if these assets were on sale then maybe I could have got it cheaper—but I didn’t find this asset until Monday. Maybe I should have looked through the Asset Store earlier. Then again, even if these assets were 50% off during the sale (which I don’t know if they were, or if they were included in the sale at all), that still would have been more than forty dollars, which is still a significant chunk of change for me right now.
So, anyway, I guess if I want to find assets I like for the player and the human NPCs, at this point it looks like I have three options:
- Pay $90 for the Chibi packs from Meshtint Studios
- Suck it up and use assets that don’t look anything like what I’d envisioned
- Make my own assets
Were it not for COVID-19 having played havoc with my finances, I’d almost certainly go with option 1. As it is, option 2 is probably the most practical. But, well, I don’t like it. Realistically, I should just go ahead and live with using assets that aren’t really close to what I had in mind, but, uh, I really don’t want to.
Which leaves option 3, the stupidest option of all. I have no idea how to create my own character model for Unity, and even if I did I almost certainly wouldn’t have time before the end of the class. Or at least, that’s what part of me is trying to tell the rest of me, but the rest of me is firmly in denial.
There are, of course, courses that cover this. As a matter of fact, I think when I finish the Beginner Design course, I’ll tackle the Beginner Art course on Unity Learn next. But it looks like the Beginner Art course just covers concept art, at least as far as characters are concerned, and actually creating character models isn’t covered till the Intermediate Art course. Even then, the course lessons use ZBrush and Maya, which I don’t have and have never used; if I were to create my own character models, I’d want to use Blender, which is free and with which I have some experience. (Yes, there are free trials available for both ZBrush and Maya, but it doesn’t seem like a great idea to put a lot of time into learning programs that I’ll only be able to use for 30 days without shelling out a lot more money than I can currently afford.) Still, that’s not a complete deal-killer; I could probably figure out how to do things in Blender well enough to follow along with the course. More pertinent is the fact that it seems very unlikely I’d be able to complete the courses before the Graduation Play-a-thon, let alone in time to put their content into practice and actually create models.
But there are other resources besides Unity Learn. After a little websearching, I found an online tutorial on another site that focuses specifically on using Blender to create and rig a simple human character for use in Unity with the built-in Unity Humanoid Avatar… which is exactly what I need. So yeah, at some point in the next few days I’ll try to use that tutorial to make some models for the player, the priestess, and maybe an additional NPC or two. As with the current UI graphics, these won’t be final versions; they’ll be placeholders that I can replace with more polished versions later, after the Create With Code course is over. But at least they should give a much better sense of what the game’s supposed to look like than the capsule primitive that represents the player now.
So, yeah. Ideally, within the next week, I hope to finish the Beginner Design course on Unity Learn, implement NPCs and conversations in Last Day to Live, replace the enemy and environment primitives with assets from the Asset Store, and create my own models for the player and a few human NPCs. Oh, and maybe implement interactable items as well. Okay, so I’ve got a lot of work ahead of me…